In Android, you can use “android.widget.ImageButton” to display a normal “
Button
“, with a customized background image.In this tutorial, we show you how to display a button with a background image named “android_button.png“, when user click on it, display a short message. As simple as that.
Note
You may also like this Android ImageButton selector example, which allow to change button’s images depends on button states.
You may also like this Android ImageButton selector example, which allow to change button’s images depends on button states.
P.S This project is developed in Eclipse 3.7, and tested with Android 2.3.3.
1. Add Image to Resources
Put image “android_button.png” into “res/drawable-?dpi” folder. So that Android know where to find your image.
2. Add ImageButton
Open “res/layout/main.xml” file, add a “
ImageButton
” tag, and defined the background image via “android:src
“.File : res/layout/main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical" >
<ImageButton
android:id="@+id/imageButton1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/android_button" />
</LinearLayout>
3. Code Code
Here’s the code, add a click listener on image button.
File : MyAndroidAppActivity.java
package com.mkyong.android;
import android.app.Activity;
import android.os.Bundle;
import android.widget.ImageButton;
import android.widget.Toast;
import android.view.View;
import android.view.View.OnClickListener;
public class MyAndroidAppActivity extends Activity {
ImageButton imageButton;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
addListenerOnButton();
}
public void addListenerOnButton() {
imageButton = (ImageButton) findViewById(R.id.imageButton1);
imageButton.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View arg0) {
Toast.makeText(MyAndroidAppActivity.this,
"ImageButton is clicked!", Toast.LENGTH_SHORT).show();
}
});
}
}
4. Demo
Run the application.
1. Result, a button with a customized background image.
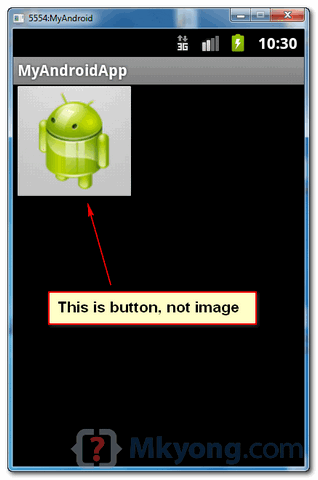
2. Click on the button, a short message is displayed.
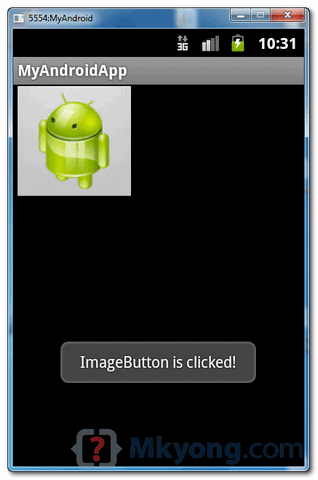
Download Source Code
Download it – Android-ImageButton-Example.zip (28 KB)
No comments:
Post a Comment